+/*
+ * Copyright (c) 2012, Oracle and/or its affiliates. All rights reserved.
+ * DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
+ *
+ * This code is free software; you can redistribute it and/or modify it
+ * under the terms of the GNU General Public License version 2 only, as
+ * published by the Free Software Foundation. Oracle designates this
+ * particular file as subject to the "Classpath" exception as provided
+ * by Oracle in the LICENSE file that accompanied this code.
+ *
+ * This code is distributed in the hope that it will be useful, but WITHOUT
+ * ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
+ * FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
+ * version 2 for more details (a copy is included in the LICENSE file that
+ * accompanied this code).
+ *
+ * You should have received a copy of the GNU General Public License version
+ * 2 along with this work; if not, write to the Free Software Foundation,
+ * Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
+ *
+ * Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
+ * or visit www.oracle.com if you need additional information or have any
+ * questions.
+ */
+package java.util;
+
+import java.util.functions.Factory;
+import java.util.functions.Mapper;
+
+/**
+ * Optional
+ *
+ * @author Brian Goetz
+ */
+public class Optional<T> {
+ private final static Optional<?> EMPTY = new Optional<>();
+
+ private final T value;
+ private final boolean present;
+
+ public Optional(T value) {
+ this.value = value;
+ this.present = true;
+ }
+
+ private Optional() {
+ this.value = null;
+ this.present = false;
+ }
+
+ public static<T> Optional<T> empty() {
+ return (Optional<T>) EMPTY;
+ }
+
+ public T get() {
+ if (!present)
+ throw new NoSuchElementException();
+ return value;
+ }
+
+ public boolean isPresent() {
+ return present;
+ }
+
+ public T orElse(T other) {
+ return present ? value : other;
+ }
+
+ public T orElse(Factory<T> other) {
+ return present ? value : other.make();
+ }
+
+ public<V extends Throwable> T orElseThrow(Factory<V> exceptionFactory) throws V {
+ if (present)
+ return value;
+ else
+ throw exceptionFactory.make();
+ }
+
+ public<V extends Throwable> T orElseThrow(Class<V> exceptionClass) throws V {
+ if (present)
+ return value;
+ else
+ try {
+ throw exceptionClass.newInstance();
+ }
+ catch (InstantiationException | IllegalAccessException e) {
+ throw new IllegalStateException("Unexpected exception: " + e, e);
+ }
+ }
+
+ public<V> Optional<V> map(Mapper<T, V> mapper) {
+ return present ? new Optional<>(mapper.map(value)) : Optional.<V>empty();
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+
+ Optional optional = (Optional) o;
+
+ if (present != optional.present) return false;
+ if (value != null ? !value.equals(optional.value) : optional.value != null) return false;
+
+ return true;
+ }
+
+ @Override
+ public int hashCode() {
+ int result = value != null ? value.hashCode() : 0;
+ result = 31 * result + (present ? 1 : 0);
+ return result;
+ }
+}
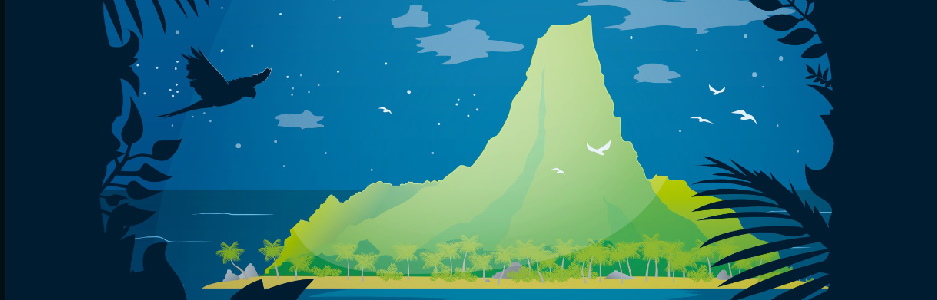